📘 قراءة كتاب Programming Visual Basic .NET أونلاين


.................................................................................................................................. 9
Organization of This Book........................................................................................ 9
Conventions Used in This Book.............................................................................. 9
How to Contact Us .................................................................................................... 10
Acknowledgments ..................................................................................................... 11
Chapter 1. Introduction..................................................................................................... 13
1.1 What Is the Microsoft .NET Framework?.................................................. 13
1.2 What Is Visual Basic .NET?............................................................................ 14
1.3 An Example Visual Basic .NET Program ................................................... 14
Chapter 2. The Visual Basic .NET Language............................................................... 23
2.1 Source Files ......................................................................................................... 23
2.2 Identifiers ............................................................................................................. 23
2.3 Keywords .............................................................................................................. 24
2.4 Literals ................................................................................................................... 27
2.5 Types...................................................................................................................... 31
2.6 Namespaces ........................................................................................................ 40
2.7 Symbolic Constants .......................................................................................... 42
2.8 Variables ............................................................................................................... 43
2.9 Scope...................................................................................................................... 44
2.10 Access Modifiers .............................................................................................. 44
2.11 Assignment........................................................................................................ 45
2.12 Operators and Expressions ......................................................................... 46
2.13 Statements........................................................................................................ 52
2.14 Classes ................................................................................................................ 60
2.15 Interfaces........................................................................................................... 85
2.16 Structures .......................................................................................................... 88
2.17 Enumerations ................................................................................................... 91
2.18 Exceptions.......................................................................................................... 93
2.19 Delegates ........................................................................................................... 98
2.20 Events................................................................................................................ 101
2.21 Standard Modules......................................................................................... 104
2.22 Attributes ......................................................................................................... 104
2.23 Conditional Compilation ............................................................................. 108
2.24 Summary.......................................................................................................... 109
Chapter 3. The .NET Framework.................................................................................. 111
3.1 Common Language Infrastructure (CLI) and Common Language
Runtime (CLR) .......................................................................................................... 111
3.2 Common Type System (CTS) ..................................................................... 111
3.3 Portions of the CLI .......................................................................................... 112
3.4 Modules and Assemblies............................................................................... 113
3.5 Application Domains....................................................................................... 116
3.6 Common Language Specification (CLS) ................................................. 116
3.7 Intermediate Language (IL) and Just-In-Time (JIT) Compilation 117
3.8 Metadata............................................................................................................. 117
3.9 Memory Management and Garbage Collection.................................... 118
3.10 A Brief Tour of the .NET Framework Namespaces........................... 122
Programming Visual Basic .NET
3
3.11 Configuration...................................................................................................125
3.12 Summary ..........................................................................................................131
Chapter 4. Windows Forms I: Developing Desktop Applications.............................133
4.1 Creating a Form................................................................................................133
4.2 Handling Form Events....................................................................................143
4.3 Relationships Between Forms .....................................................................145
4.4 MDI Applications...............................................................................................147
4.5 Component Attributes....................................................................................155
4.6 2-D Graphics Programming with GDI+...................................................160
4.7 Printing.................................................................................................................174
4.8 Summary.............................................................................................................186
Chapter 5. Windows Forms II: Controls, Common Dialog Boxes, and Menus ......187
5.1 Common Controls and Components.........................................................187
5.2 Control Events...................................................................................................204
5.3 Form and Control Layout..............................................................................204
5.4 Common Dialog Boxes...................................................................................210
5.5 Menus ...................................................................................................................215
5.6 Creating a Control ...........................................................................................227
5.7 Summary.............................................................................................................236
Chapter 6. ASP.NET and Web Forms: Developing Browser-Based Applications 237
6.1 Creating a Web Form .....................................................................................238
6.2 Handling Page Events.....................................................................................251
6.3 More About Server Controls ........................................................................253
6.4 Adding Validation.............................................................................................268
6.5 Using Directives to Modify Web Page Compilation .............................283
6.6 ASP.NET Objects: Interacting with the Framework ...........................291
6.7 Discovering Browser Capabilities...............................................................296
6.8 Maintaining State.............................................................................................298
6.9 Application-Level Code and global.asax .................................................304
6.10 Web-Application Security ...........................................................................307
6.11 Designing Custom Controls .......................................................................320
6.12 Summary ..........................................................................................................328
Chapter 7. Web Services................................................................................................329
7.1 Creating a Web Service.................................................................................329
7.2 Testing a Web Service with a Browser....................................................333
7.3 Web-Service Descriptions.............................................................................335
7.4 Consuming a Web Service............................................................................335
7.5 Web-Service Discovery..................................................................................340
7.6 Limitations of Web Services ........................................................................340
7.7 Summary.............................................................................................................341
Chapter 8. ADO.NET: Developing Database Applications .......................................343
8.1 A Brief History of Universal Data Access ................................................343
8.2 Managed Providers..........................................................................................343
8.3 Connecting to a SQL Server Database ....................................................344
SQL Server Authentication.............................................................................................347
8.4 Connecting to an OLE DB Data Source ...................................................348
8.5 Reading Data into a DataSet.......................................................................349
4
8.6 Relations Between DataTables in a DataSet ........................................ 360
8.7 The DataSet's XML Capabilities ................................................................. 362
8.8 Binding a DataSet to a Windows Forms DataGrid.............................. 364
8.9 Binding a DataSet to a Web Forms DataGrid....................................... 367
8.10 Typed DataSets ............................................................................................. 368
8.11 Reading Data Using a DataReader......................................................... 370
8.12 Executing Stored ProceduresThrough a SqlCommand Object.... 371
8.13 Summary.......................................................................................................... 374
Appendix A. Custom Attributes Defined in the System Namespace ...................... 375
Appendix B. Exceptions Defined in the System Namespace................................... 381
Appendix D. Resources for Developers ...................................................................... 391
D.1 .NET Information............................................................................................. 391
D.2 Discussion Lists................................................................................................ 392
Netiquette ......................................................................................................................... 392
Appendix E. Math Functions.......................................................................................... 395
Colophon....
Explain application for Visual Basic PDF
visual basic 2010 pdf شرح
فيجوال بيسك pdf
visual basic codes list pdf
فيجوال بيسك 6 شرح
visual basic 6.0 programming examples pdf
visual basic codes for beginners
تعليم فيجوال بيسك 6 خطوة خطوة حتى الإحتراف pdf
visual basic net pdf
سنة النشر : 2012م / 1433هـ .
حجم الكتاب عند التحميل : 2.5 ميجا بايت .
نوع الكتاب : pdf.
عداد القراءة:
اذا اعجبك الكتاب فضلاً اضغط على أعجبني و يمكنك تحميله من هنا:
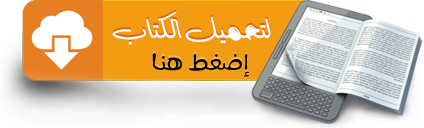
شكرًا لمساهمتكم
شكراً لمساهمتكم معنا في الإرتقاء بمستوى المكتبة ، يمكنكم االتبليغ عن اخطاء او سوء اختيار للكتب وتصنيفها ومحتواها ، أو كتاب يُمنع نشره ، او محمي بحقوق طبع ونشر ، فضلاً قم بالتبليغ عن الكتاب المُخالف:
قبل تحميل الكتاب ..
يجب ان يتوفر لديكم برنامج تشغيل وقراءة ملفات pdf
يمكن تحميلة من هنا 'http://get.adobe.com/reader/'