📘 قراءة كتاب C Programming Tutorial أونلاين


xi
1 Introduction
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
1
1.1 High Levels and Low Levels
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
1
1.2 Basic ideas about C
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
5
1.3 The Compiler
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
5
1.4 Errors
.. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
. . .
8
1.5 Use of Upper and Lower Case
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
9
1.6 Declarations
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
10
1.7 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
10
2 Reserved words and an example
. . . . . . . . . . .
11
2.1 The
printf()
function
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
11
2.2 Example Listing
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
12
2.3 Output
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
.
12
2.4 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
12
3 Operating systems and environments
. . . . . .
13
3.1 Files and Devices
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
13
3.2 Filenames
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
14
3.3 Command Languages and Consoles
. . . . . . . . . . . . . . . . . . . . . . . . . .
14
3.4 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
15
4 Libraries
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
17
4.1 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
19
5 Programming style
. . . . . . . . . . . . . . . . . . . . . . .
21
6 The form of a C program
. . . . . . . . . . . . . . . . .
23
6.1 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
26
7 Comments
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
27
7.1 Example 1
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
27
7.2 Example 2
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
27
7.3 Question
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
28
ii
8 Functions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
29
8.1 Structure diagram
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
31
8.2 Program Listing
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
31
8.3 Functions with values
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
32
8.4 Breaking out early
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
34
8.5 The
exit()
function
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
34
8.6 Functions and Types
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
35
8.7 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
35
9 Variables, Types and Declarations
. . . . . . . . .
37
9.1 Declarations
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
38
9.2 Where to declare things
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
38
9.3 Declarations and Initialization
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
39
9.4 Individual Types
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
40
9.4.1
char
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
40
9.4.2 Listing
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
41
9.4.3 Integers
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
42
9.5 Whole numbers
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
42
9.5.1 Floating Point
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
42
9.6 Choosing Variables
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
43
9.7 Assigning variables to one another
. . . . . . . . . . . . . . . . . . . . . . . . . . .
44
9.8 Types and The Cast Operator
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
44
9.9 Storage class
static
and
extern
. . . . . . . . . . . . . . . . . . . . . . . . . . . .
47
9.10 Functions, Types and Declarations
. . . . . . . . . . . . . . . . . . . . . . . . .
48
9.11 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
49
10 Parameters and Functions
. . . . . . . . . . . . . . . .
51
10.1 Declaring Parameters
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
51
10.2 Value Parameters
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
52
10.3 Functions as actual parameters
. . . . . . . . . . . . . . . . . . . . . . . . . . . . .
57
10.4 Example Listing
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
57
10.5 Example Listing
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
58
10.6 Variable Parameters
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
60
10.7 Example Listing
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
63
10.8 Questions
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
63
11 Scope : Local And Global
. . . . . . . . . . . . . . . .
65
11.1 Global Variables
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
65
11.2 Local Variables
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
65
11.3 Communication : parameters
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
68
11.4 Example Listing
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
68
11.5 Style Note
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
69
11.6 Scope and Style
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
70
11.7 Questions
. . ook.
نوع الكتاب : zip.
عداد القراءة:
اذا اعجبك الكتاب فضلاً اضغط على أعجبني و يمكنك تحميله من هنا:
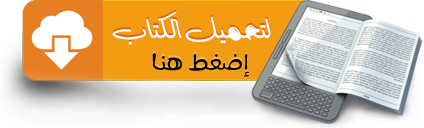
شكرًا لمساهمتكم
شكراً لمساهمتكم معنا في الإرتقاء بمستوى المكتبة ، يمكنكم االتبليغ عن اخطاء او سوء اختيار للكتب وتصنيفها ومحتواها ، أو كتاب يُمنع نشره ، او محمي بحقوق طبع ونشر ، فضلاً قم بالتبليغ عن الكتاب المُخالف:
قبل تحميل الكتاب ..
يجب ان يتوفر لديكم برنامج تشغيل وقراءة ملفات pdf
يمكن تحميلة من هنا 'http://get.adobe.com/reader/'